728x90
반복자 패턴은 컬렉션의 노출을 막으면서 컬렉션의 요소를 탐색 할 수 있는 패턴이다.
객체 안에 들어있는 모든 항목에 접근하는 방식이 통일되어 있으면 어떤 종류의 컬렉션에 대해서도 사용할 수 있다.
=> 모든 컬렉션은 이터렉터를 구현되어 있다는 뜻!
이 패턴을 사용하면 모든 항목에 일일이 접근하는 작업을 컬렉션 객체가 아닌 반복자 객체에서 맡게된다. 그리하면 집합체의 인터페이스 및 구현이 간단해질 뿐만 아니라 집합체에서는 반복작업에서 손을 떼고 월래 자신이 할일(객체 컬렉션 관리)에만 전념할 수 있다.
구조
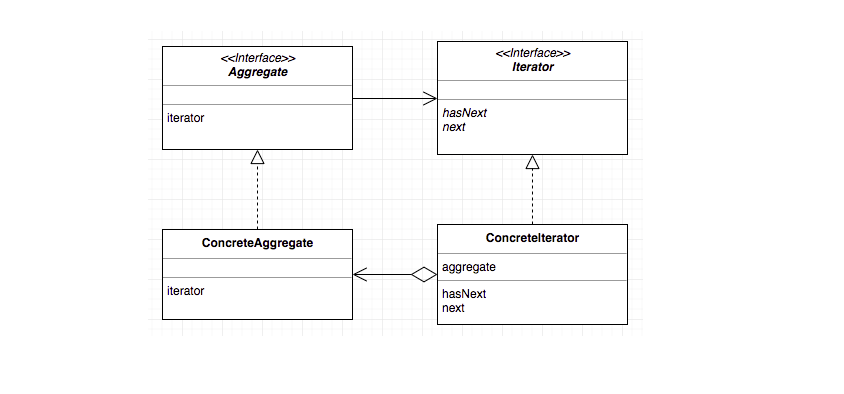
▷ Aggregate : Iterator, 다음 요소를 가지오는, 현재 위치 검색 등을 만들어내는 인터페이스이다.
▷ Iterator : 요소를 순서대로 검색해가는 인터페이스이다. 다음 요소가 존재하는지 hasNext와 next 메소드를 통해 결정한다.
▷ ConcreteAggregate : Aggregate 인터페이스의 구현체. 컬렉션을 순회하기 위한 알고리즘 구현
▷ ConcreteIterator : Iterator 인터페이스의 구현체
구현
public interface Aggregate
{
Iterator iterator();
}
public interface Iterator
{
bool hasNext();
object next();
}
======================================
public class Book
{
private string name;
public Book(string name)
{
this.name = name;
}
public string GetName()
{
return this.name;
}
}
======================================
public class BookShelf : Aggregate
{
private Book[] books;
private int last = 0;
public BookShelf(int size)
{
this.books = new Book[size];
}
public Book GetBookAt(int index)
{
return books[index];
}
public void AppendBook(Book book)
{
this.books[last] = book;
last++;
}
public int GetLength()
{
return last;
}
public Iterator iterator()
{
return new BookShelfIterator(this);
}
}
======================================
public class BookShelfIterator : Iterator
{
private BookShelf bookShelf;
private int index;
public BookShelfIterator(BookShelf bookShelf)
{
this.bookShelf = bookShelf;
this.index = 0;
}
public bool hasNext()
{
if (index < bookShelf.GetLength())
{
return true;
}
else
{
return false;
}
}
public object next()
{
Book book = bookShelf.GetBookAt(index);
index++;
return book;
}
}
======================================
static void Main(string[] args)
{
BookShelf bookShelf = new BookShelf(4);
bookShelf.AppendBook(new Book("Around the World in 80 Days"));
bookShelf.AppendBook(new Book("Bible"));
bookShelf.AppendBook(new Book("Cinderella"));
bookShelf.AppendBook(new Book("Baddy-Long-Legs"));
Iterator it = bookShelf.iterator();
while (it.hasNext())
{
Book book = (Book)it.next();
Console.WriteLine(book.GetName());
}
Console.ReadLine();
}
728x90
'STUDY > Design Pattern' 카테고리의 다른 글
해석자 패턴(Interpreter pattern) (0) | 2022.12.28 |
---|---|
명령패턴 (Command Pattern) (0) | 2022.12.28 |
책임 연쇄 패턴(Chain of Responsibility Pattern) (0) | 2022.12.28 |
프록시 패턴(Proxy Pattern) (0) | 2022.12.19 |
경량 패턴(Flyweight Pattern) (0) | 2022.12.19 |